Angular 17 introduced a new template syntax called @switch
, that allows to show or hide template sections depending on a logical condition.
This new syntax is an alternative to the previous ngSwitch
structural directive.
In this guide, I will explain the @switch
template syntax and how to use it in your Angular application.
Table of Contents
This post covers the following topics:
- What is
@switch
? - Using
@switch
with multiple@case
blocks - The
@default
clause in@switch
ngSwitch
vs@switch
- Migrating to
@switch
using the Angular CLI - Summary
Note: If you're looking for the @if
or @for
syntaxes, check out my other guides:
You can read all about @switch
and the new template syntax in the official docs: Angular Control Flow.
What is @switch
?
The @switch
syntax is for Angular what the switch
statement is for Javascript.
The @switch
template syntax is used to select the right template section to render based on the value of an expression.
Typically you want to use @switch
when you have at least 2 or more different options to display, depending on the value of the expression.
If on the other hand you only have two options to display, you usually want to use the @if
syntax instead.
Notice that the @switch
template syntax is not a directive. This feature is built right into the template engine, just like @if
or @for
.
Here is how it works.
Using @switch
with multiple @case
blocks
Let's see an example of @switch
in action:
@switch (color) {
@case ("red") {
<div>Red</div>
}
@case ("blue") {
<div>Blue</div>
}
}
The code above will render:
- "Red" if the value of the
color
variable is "red" - "Blue" if the value of the
color
variable is "blue"
You can see the similarities between @switch
and the Javascript switch statement:
switch (color) {
case "red":
// code
break;
case "blue":
// code
break;
}
The @default
clause in @switch
The @default
clause is used to render a template when none of the @case
blocks matches the value of the expression.
Let's see an example:
@Component({
template: ` @switch (color) {
@case ("red") {
<div>Red</div>
}
@case ("blue") {
<div>Blue</div>
}
@default {
<div>Default</div>
} }`,
})
export class AppComponent {
color = "green";
}
The code above will render "Default" because none of the @case
blocks matches the value "green" of the color variable.
ngSwitch
vs @switch
The @switch
syntax is a direct replacement for the ngSwitch
structural directive.
It has the following benefits over the ngSwitch
directive:
-
it's much more intuitive, it reads just like a plain switch statement in Javascript
-
no explicit imports are needed in standalone components. The
@switch
syntax is automatically available in all templates, unlikengSwitch
Migrating to @switch
using the Angular CLI
The Angular CLI has a command that can be used to migrate from ngSwitch
to @switch
:
ng generate @angular/core:control-flow
This command will migrate all the *ngSwitch
directives in your project to the new syntax, and it will do the same for @if
and @for
.
I hope you enjoyed this post, to get notified when new similar posts like this are out, I invite you to subscribe to our newsletter:
You will also get up-to-date news on the Angular ecosystem.
And if you want a deep dive into all the features of Angular Core like @switch
, have a look at the Angular Core Deep Dive Course:
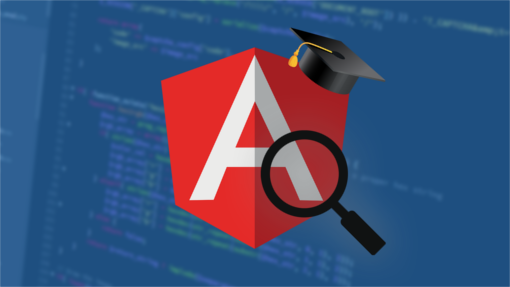
Summary
In this guide, we explored the new @switch
template syntax in detail.
As you can see, it's super intuitive and it reads just like a plain switch statement in Javascript.
No imports are needed, and you can easily migrate to it using the Angular CLI.
So go ahead and try it out, and let me know in the comments below of any questions you might have!