The Angular road to full application-wide signal-based reactivity continues with the latest Angular 17.1 release.
We just made one major step forward with the inclusion of Signal Inputs in 17.1, and besides that, we have a few other interesting features that we are going to cover in this post.
So without further ado, let's get started!
Table of Contents
- Input Signals
- New Router info parameter
- New Test Runner
- Improved control flow migration
- Application builder migration
- Development server shortcuts
- Angular Material support for hydration
- Typescript and Vite upgrades
- Summary
Input Signals
By far the biggest feature that has been released in Angular 17.1 is signal inputs.
In this post, we are just going to give you the gist of signal inputs, but if you want a deep dive into Angular Signals, check out this other article I wrote: Angular Signal Inputs.
A signal input is a new signal-based alternative to the traditional @Input
decorator.
Here is what a signal input looks like:
@Component({
selector: "counter",
standalone: true,
template: ` <h1>Counter value: {{ value() }}</h1>`,
})
export class CounterComponent {
value = input(0);
}
Our input value is now a signal! So we finally have a reactive component input primitive in Angular.
Notice in the template that to access the actual value of the signal, we need to invoke the signal as a function using ()
.
To learn more about signal inputs, check this video on the Angular University YouTube Channel:
New Router info parameter
Next up, we have available a new advanced router option called the info
parameter.
Here is how we can use it:
<button (click)="onIncrement()">
Increment
</button>
<button
routerLink="home"
[info]="{hello: 'world'}">
Navigate to Home
</button>
So now in a router navigation, you can add an extra transient info
parameter object.
This object is going to be part of the NavigationExtras object.
You can retrieve it in the following way:
@Component({...})
export class HomeComponent {
constructor(private router: Router) {
}
ngOnInit() {
this.router.events
.pipe(
filter((e) => e instanceof NavigationEnd),
map((e) =>
this.router
.getCurrentNavigation()
?.extras.info)
)
.subscribe((info) => {
console.log(info);
});
}
}
Notice that you can pass the info parameter either programmatically or by using the declarative router link API.
By getting the current navigation and from the extras object, you can grab the info
parameter.
Notice that this info
parameter is not being persisted in the browser history API, so if you hit the back-and-forth button on the browser, it's not going to be reflected in the URL.
For example, if you click on the Navigate to Home
button, you're going to notice that nothing shows up in the URL, so the info
extra parameter is not persisted in the history stack.
If you hit back and forward again, the info
parameter is not going to be passed.
The info
extra parameter is purely transient information that you might want to use in certain situations.
Most of the time, this is not a feature that you would be using every single day, but if you ever need to pass some extra information in specific route navigation without that information getting persisted in the URL, now there is a way to do it using a standard Angular API.
New Web Test Runner
Next up is the new Web Test Runner that you now have available.
This browser test runner is in principle going to be the future replacement of Karma, which is now over 10 years old.
If you want to already start using this Web Test Runner, you need to install the following package in your project:
npm install @web/testrunner --save-dev
The test runner itself is production-ready, but its integration with Angular is still experimental.
You can replace Karma with this test runner in the following way:
- Go to your
angular.json
file. - Go to your
builder
configuration for your test task.
"test": {
"builder": "@angular-devkit/build-angular:karma",
"options": {
"main": "src/test.ts",
"karmaConfig": "./karma.conf.js",
"polyfills": "src/polyfills.ts",
"tsConfig": "src/tsconfig.spec.json",
"scripts": [],
"styles": [
"src/styles.scss"
],
"assets": [
"src/assets",
"src/favicon.ico"
]
}
},
- Here, instead of
karma
. Simply use theweb-test-runner
in the following way:web-test-runner
.
"test": {
"builder": "@angular-devkit/build-angular:web-test-runner",
"options": {
"main": "src/test.ts",
"karmaConfig": "./karma.conf.js",
"polyfills": "src/polyfills.ts",
"tsConfig": "src/tsconfig.spec.json",
"scripts": [],
"styles": [
"src/styles.scss"
],
"assets": [
"src/assets",
"src/favicon.ico"
]
}
},
Now, if you run your tests here in your command line using the usual command ng test
, you are going to see that the new Web Test Runner is being used!
Improved control flow migration
Next up, we have several improvements on the Angular CLI migrations.
The existing control flow migration is now updated and improved.
A lot of bugs were fixed, and new features were added, so if you want to migrate your projects from *ngFor
, *ngIf
, *ngSwitch
to the new control flow syntax: @if
, @for
, @switch
, you can run this migration:
ng generate @angular/core:control-flow
Notice that the migration itself is still on developer preview, but this new version is much improved, so we might want to try it out.
Application builder migration
Still on the topic of Angular CLI migrations, if you want to upgrade your existing legacy project to use the new application Builder.
You have a new migration available, and your angular.json
file is going to be updated to use the new application Builder:
ng update @angular/cli --name =use-application-builder
New Angular CLI Development server shortcuts
Next up, there are a couple of nice developer experience improvements in the Angular CLI development server.
Notice this new message on the Angular CLI developer server:
Application bundle generation complete. [0.281 seconds]
Watch mode enabled. Watching for file changes...
Local: http://localhost:4200/
press + enter to show help
If you press H
plus Enter
, you will have a couple of useful shortcuts that we didn't have available before.
-
r + enter
: to force the reload of the browser, -
u + enter
: to show the server URL that we are currently running, -
o + enter
: to open a new window in the browser with the Angular CLI development server -
c + enter
: to clear the console and -
q + enter
: to quit. -
So go ahead and try out the new shortcuts, it's a very nice improvement on the developer experience!
Angular Material support for SSR client hydration
Next up, we have some important improvements in the Angular material Library.
Now all the Angular material components are fully Standalone, and also they support server-side rendering client hydration.
This means that if you are building your application using Angular Material, your front end will no longer re-render everything that the server has just rendered if you are using client-side hydration, which is recommended.
Go ahead and check out a video that I have here on the channel: The Angular SSR Deep dive, where I explain in detail client hydration and how server-side rendering works in general.
Typescript and Vite upgrades
We also have an upgrade to TypeScript 5.3 and to Vite 5 at the level of the Angular CLI build.
And that's it for the 17.1 release, not bad right?
If you want to get notified when new Angular releases are out and get a comprehensive summary like this one each time by email, I invite you to subscribe to my newsletter:
You will also get up-to-date news on the Angular ecosystem.
And if you want a deep dive into all the features of Angular Core like Signals
and others, have a look at the Angular Core Deep Dive Course:
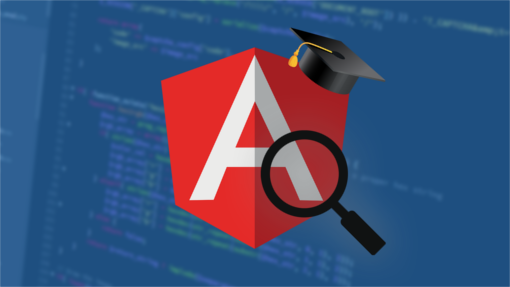
Summary
We have seen in this post a quick summary of all the eight major new features of Angular 17.1 that are just out.
The major feature is signal inputs, which is a new signal-based alternative to the traditional @Input
decorator.
This is a major step forward in the whole Angular signal-based reactivity story, and I anticipate that we are going to see more and more of these signal-based features in the near future!
Let me know if you have any questions or comments, I'll be happy to help!